Randomization is an essential element in game development that can enhance the gaming experience by introducing unpredictability and variety. There are several ways you can do Random Numbers In Unity. and mastering the art of randomization can be challenging, and game developers must have a deep understanding of the various techniques and tools available.
This article aims to provide game developers with practical tips and tricks for mastering randomization in Unity. The article will explore different methods for generating random values, such as using the Random Range function, as well as more advanced techniques, such as randomizing vector positions, colors, and rotations.
Additionally, the article will provide guidance on using random numbers for probability and introduce resources that can help developers further develop their skills in randomization.
By the end of the article, game developers should have a solid understanding of how to use randomization to create unique and engaging gameplay experiences in Unity.
Random Numbers in Unity Key Takeaways
- Randomization is important for creating varied gameplay and behaviors in games.
- Unity’s Random Class is Static, meaning any script can access the same set of random values.
- Randomizing colours in Unity can be done by assigning a random value between 0 and 1 to each parameter of a new Color variable.
- Weighted random decisions in Unity involve options that are more or less likely to be picked based on their weight relative to the other options.
Randomizing Gameplay
Randomizing gameplay in Unity can be achieved through various methods such as generating random positions, rotations, and colors using the Random Class, as well as creating weighted random decisions for loot drops or selecting options from a list.
Randomizing enemy behavior is essential for creating varied gameplay experiences. By using random values to determine enemy movements, attacks, or behaviors, game developers can increase the replayability of their games. For example, an enemy that moves randomly instead of following a predictable pattern can offer a more challenging and engaging experience for players.
Implementing random events in game levels is another way to create varied gameplay. Random events can include spawning enemies at random locations, generating new obstacles, or triggering different paths to take. Random events can add an element of surprise to the game and keep players on their toes.
However, it’s important to ensure that random events are balanced and not too frequent, as they can become frustrating for players if they occur too often.
Overall, randomizing gameplay can add an exciting and unpredictable factor to games, making them more enjoyable for players.
Generating Random Values
Generating varied gameplay and behaviors in games requires the use of random values, which can be achieved through various methods such as randomizing positions and rotations, generating random colors, and creating weighted decisions. Randomizing input controls is another way to add variability to gameplay. By using random values to alter the behavior of input controls, players can experience a unique gaming experience each time they play. This can be achieved by adding random delays, altering the sensitivity of controls, or randomly swapping the functions of different buttons.
Implementing random sound effects is another way to add variety to games. Random sound effects can be used to create a sense of unpredictability and excitement in gameplay. By using random values to trigger different sound effects, players can experience a more immersive gaming experience. This can be achieved by using a script to randomly select from a pool of sound effects or by using a random number generator to determine which sound effect to play.
using UnityEngine;
public class RandomGenerator : MonoBehaviour
{
private void Start()
{
// Generate a random integer between 1 and 10 (inclusive)
int randomInt = Random.Range(1, 11);
Debug.Log("Random Integer: " + randomInt);
// Generate a random float between 0.0f and 1.0f
float randomFloat = Random.value;
Debug.Log("Random Float: " + randomFloat);
// Generate a random position in the game world
Vector3 randomPosition = new Vector3(Random.Range(-10f, 10f), Random.Range(0f, 5f), Random.Range(-10f, 10f));
Debug.Log("Random Position: " + randomPosition);
}
}
This script demonstrates three common ways to generate random values in Unity using the Random
class: generating a random integer within a range, generating a random float between 0.0 and 1.0, and generating a random position in the game world.
You can attach this script to any GameObject in your scene to see the generated values in the Unity Editor console when you play the game.
From an overall point of view, the use of random values in game development can add a layer of unpredictability and excitement to gameplay, making the gaming experience more enjoyable for players.
Using Random Numbers for Probability
The use of random numbers for probability is a common technique in game development that allows for the creation of dynamic and unpredictable gameplay.
Unity’s Random Value function allows developers to generate a random float between 0 and 1, which can be used to determine the probability of a certain event occurring. For example, a developer could use Random Value to randomly determine whether an enemy AI will attack the player, with a 50% chance of attacking and a 50% chance of staying idle.
float randomValue = UnityEngine.Random.value;
Randomizing AI behavior can add an element of surprise to gameplay, keeping players engaged and on their toes. Additionally, randomization can be used to create variation in sound effects, such as randomizing the pitch or volume of a gunshot or explosion. This can make the game world feel more alive and immersive, as no two instances of an effect will sound exactly the same.
Randomizing AI Behavior
using UnityEngine;
public class AIBehavior : MonoBehaviour
{
// List of possible behaviors or actions for the AI
public string[] behaviors;
private void Start()
{
// Call a method to randomize AI behavior on start
RandomizeBehavior();
}
private void Update()
{
// Check if certain condition is met, then call the method to randomize AI behavior again
if (/* Insert your condition here */)
{
RandomizeBehavior();
}
}
private void RandomizeBehavior()
{
// Generate a random index within the range of available behaviors
int randomIndex = Random.Range(0, behaviors.Length);
// Retrieve the randomly selected behavior from the array and perform it
string currentBehavior = behaviors[randomIndex];
// Implement your logic based on the current behavior, e.g., move, attack, etc.
Debug.Log("AI is now performing: " + currentBehavior);
}
}
To use this code in Unity, create a new C# script called AIBehavior
and attach it to your AI game object. Make sure you have an array of possible behaviors defined (behaviors
) and fill it with different actions or states you want your AI to exhibit.
Remember to replace /* Insert your condition here */
with the specific condition that triggers a change in AI behavior. For example, if you want the AI behavior to change randomly every few seconds, you can use Time.time % 3 == 0
as a simple condition.
By employing this code, your AI will randomly select a behavior from the given array and perform it. This randomness will add an element of surprise to gameplay as the AI’s actions will be unpredictable.
Randomizing Sound effects
using UnityEngine;
public class RandomSoundEffect : MonoBehaviour
{
public AudioClip[] soundEffects; // Array to hold the sound effect clips
private AudioSource audioSource;
void Start()
{
audioSource = GetComponent<AudioSource>();
// Play a random sound effect on start
PlayRandomSoundEffect();
}
void PlayRandomSoundEffect()
{
if (soundEffects.Length > 0)
{
// Generate a random index within the range of the soundEffects array
int randomIndex = Random.Range(0, soundEffects.Length);
// Play the randomly selected sound effect
audioSource.clip = soundEffects[randomIndex];
audioSource.Play();
}
}
}
n this example, we have a RandomSoundEffect
script attached to a GameObject in Unity. The script includes an array called soundEffects
which holds various AudioClip objects representing different sound effects.
The Start()
function sets up the AudioSource component and calls the PlayRandomSoundEffect()
function to play a random sound effect when the game starts.
The PlayRandomSoundEffect()
function generates a random index within the range of the soundEffects
array and then plays the corresponding sound effect using an AudioSource component.
Remember to attach this script to a GameObject with an AudioSource component, assign your desired sound effects to the soundEffects
array in the Unity editor, and make any necessary adjustments to fit your specific project requirements.
Developers can use Unity’s Random Range function to generate random values for these effects, allowing for a wide range of possibilities. By incorporating randomization into various aspects of game development, developers can create a more dynamic and engaging experience for players.
Creating Random Colors and Positions
Creating unique and diverse visuals in a game can be achieved by using random values to determine colors and positions, providing a sense of unpredictability and variety. Unity offers various methods of generating random colors, including randomizing RGB values or using the built-in Random Color HSV function. Hue, saturation, and value (brightness) are the elements of HSV colors in Unity. By excluding or limiting some parameters of the HSV color value, random colors can be created within controlled limits. Randomizing just the hue can create vivid, bright colors, while limiting the saturation and brightness can create more muted tones.
using UnityEngine;
public class RandomColorsAndPositions : MonoBehaviour
{
public GameObject prefab;
public int numberOfObjects = 10;
public float minX = -5f;
public float maxX = 5f;
public float minY = -5f;
public float maxY = 5f;
void Start()
{
GenerateObjects();
}
void GenerateObjects()
{
for (int i = 0; i < numberOfObjects; i++)
{
// Instantiate the object from the prefab
GameObject obj = Instantiate(prefab);
// Set a random color
Renderer renderer = obj.GetComponent<Renderer>();
renderer.material.color = Random.ColorHSV();
// Set a random position within the specified range
Vector3 randomPosition = new Vector3(Random.Range(minX, maxX), Random.Range(minY, maxY), 0f);
obj.transform.position = randomPosition;
}
}
}
To use this script, follow these steps:
- Create an empty
GameObject
in your Unity scene. - Attach this script to the empty
GameObject
. - Create or import a game object as a prefab (such as a cube or sphere) and assign it to the
prefab
variable in the inspector. - Specify the number of objects you want to generate with
numberOfObjects
. - Define the minimum and maximum X and Y values for positions using
minX
,maxX
,minY
, andmaxY
variables.
When you run the game, it will dynamically generate the specified number of objects with random colors and positions within the defined range.
Randomizing materials is another way to add variation to game visuals. Unity’s Random Class can be used to randomly select a material from an array of materials, which can be assigned to objects in the scene. Additionally, random sound effects can be generated using Unity’s Audio Mixer and Random Class. By assigning multiple sounds to the same audio source and using the Random Class to randomly play one of the sounds, a sense of unpredictability can be added to the game’s audio. Overall, using random values to determine colors, materials, and sounds can enhance a game’s visual and auditory experience, providing players with a sense of diversity and unpredictability.
Randomizing Materials | Randomizing Colors | Randomizing Sound Effects |
---|---|---|
Unity’s Random Class can be used to randomly select a material from an array of materials. | Unity offers various methods of generating random colors, including randomizing RGB values or using the built-in Random Color HSV function. | By assigning multiple sounds to the same audio source and using the Random Class to randomly play one of the sounds, a sense of unpredictability can be added to the game’s audio. |
Materials can be assigned to objects in the scene to add variation to game visuals. | Hue, saturation, and value (brightness) are the elements of HSV colors in Unity. | Unity’s Audio Mixer can be used to control the sound effects’ volume, pitch, and other parameters. |
Randomizing materials can be used to create a sense of unpredictability in game visuals. | Randomizing just the hue can create vivid, bright colors, while limiting the saturation and brightness can create more muted tones. | Multiple sounds can be assigned to each audio source to increase the variety of sound effects. |
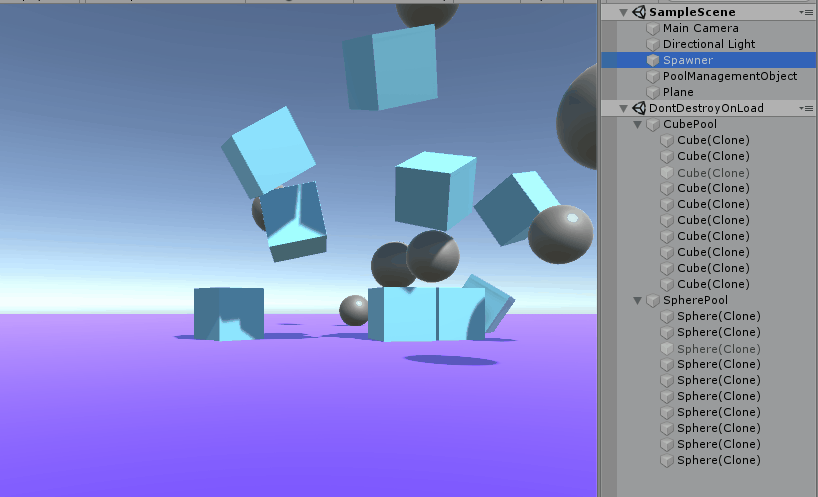
Randomizing Object / Character Spooling
Before getting into pooling of game objects, we need to explain the concept of Objct pooling.
What is GameObject Pooling in Unity game engine?
GameObject pooling is a technique used in the Unity game engine to efficiently manage and reuse GameObjects. In Unity, creating and destroying GameObjects dynamically can be resource-intensive and may cause performance issues, especially if done frequently.
Pooling involves creating a predefined number of GameObject instances upfront and reusing them throughout the gameplay instead of constantly creating and destroying new instances. This approach helps reduce the overhead associated with instantiating and destroying objects on the fly.
Here’s how it typically works:
- During initialization or at runtime, you create a pool of GameObject instances by instantiating a certain number of them.
- Instead of destroying a GameObject when it is no longer needed, you disable it or move it out of view.
- When you need to use an object from the pool again, you enable or move it back into view, reset its properties as needed, and reuse it.
- This process continues throughout the game, allowing for efficient recycling of objects without constantly creating new ones.
By reusing existing objects through pooling, you can significantly improve performance by minimizing memory allocation and garbage collection overhead. It can be particularly useful when dealing with frequently created/destroyed objects like bullets, enemies, particles, or other dynamic elements.
There are various ways to implement object pooling in Unity using techniques such as queues or custom data structures. Some developers also use specialized pooling plugins available in the Unity Asset Store for added convenience.
Overall, object pooling is an effective technique to optimize performance when dealing with dynamic GameObject instantiation and destruction in Unity games.
Here’s a table outlining the benefits and inconveniences of Object Pooling in C# Unity game engine:
Benefits | Inconveniences |
---|---|
Reusing objects instead of creating and destroying them repeatedly can improve performance by reducing memory allocation and garbage collection overhead. | Object pooling requires careful management to ensure that objects are properly initialized and reset when borrowed or returned to the pool. |
By reusing objects, you can minimize frame rate drops caused by frequent object instantiation and destruction, resulting in a smoother gameplay experience. | Implementing object pooling adds additional complexity to your codebase, potentially making it harder to maintain or understand. |
Object pooling can help alleviate CPU spikes that occur during heavy object creation, as initialization steps are performed only once when an object is first created. | It may be challenging to determine the optimal pool size and manage the dynamic nature of object usage, which requires monitoring and adjusting as needed. |
Pooling often leads to improved scalability, allowing your game to handle larger numbers of objects without sacrificing performance. | Certain types of objects may not be suitable for pooling due to their complex state or dependency on external resources. |
Remember that depending on your specific use case and requirements, the benefits and inconveniences may vary.
using UnityEngine;
public class CharacterSpawner : MonoBehaviour
{
public GameObject[] characterPrefabs; // Array of character prefabs to be instantiated
public Transform spawnPoint; // The position where characters will be spawned
private void Start()
{
SpawnCharacter();
}
private void SpawnCharacter()
{
int randomIndex = Random.Range(0, characterPrefabs.Length); // Get a random index within the range of available characters
Instantiate(characterPrefabs[randomIndex], spawnPoint.position, Quaternion.identity);
// Instantiate a character prefab at the spawnPoint with no rotation (Quaternion.identity)
}
}
In this code, you need to create an empty GameObject in your scene and attach this script to it. Also, make sure to assign the character prefabs and the spawn point in the inspector.
The characterPrefabs
array holds all the different character prefabs that you want to randomly instantiate. The SpawnCharacter()
method chooses a random index from the array using Random.Range
, then instantiates a random character prefab at the specified spawnPoint
.
You can customize this code by modifying the number of characters in your characterPrefabs
array or adjusting other aspects based on your specific needs.
Remember to attach this script to an empty GameObject in your scene and set up the necessary variables in the inspector for it to work properly.

Procedural Generation
Random numbers are often used to generate procedural content, such as terrain, levels, or maze layouts. By using random numbers as inputs to algorithms, you can create unique and unpredictable game environments.
Here’s a simple example of procedural generation in C# using the Unity Game Engine:
using UnityEngine;
public class ProceduralGeneration : MonoBehaviour
{
public int width = 10;
public int height = 10;
public GameObject prefab;
void Start()
{
GenerateLevel();
}
void GenerateLevel()
{
for (int x = 0; x < width; x++)
{
for (int y = 0; y < height; y++)
{
Vector3 position = new Vector3(x, 0, y);
Instantiate(prefab, position, Quaternion.identity);
}
}
}
}
In this example, we have a script called ProceduralGeneration
attached to an empty game object in the scene. It has three variables: width
and height
, which determine the size of the generated grid, and prefab
, which is the game object that we want to instantiate.
In the Start
method, we call the GenerateLevel
function to create our level. Inside the GenerateLevel
function, we use nested loops to iterate over each position in our grid. We create a new Vector3
representing the position of each cell and then instantiate a copy of our prefab at that position using Instantiate
.
You can attach this script to an empty game object in your Unity scene and assign a prefab to it in the inspector. When you run the game, you will see a grid of instantiated objects based on the specified dimensions.
Feel free to customize this code according to your specific needs and add additional logic for generating more complex and interesting levels!
Additional Resources
In addition to the information provided in the article, there are numerous resources available online for game developers looking to enhance their skills and knowledge in Unity and game development.
One such resource is the Unity website itself, which offers a wealth of tutorials, forums, and documentation on all aspects of game development using their platform. They also have an asset store where developers can purchase pre-made assets, tools, and plugins to aid in their game development process.
Another useful resource for Unity game developers is the Rewired input management tool. This tool simplifies the process of managing input from various devices such as controllers, keyboards, and touchscreens, allowing developers to focus more on the game’s mechanics and less on the technical details.
Similarly, Easy Save is a tool that simplifies the process of game save and file serialization management, allowing developers to easily save and load game data without needing to write complex code.
By utilizing these resources, game developers can streamline their development process and focus more on creating engaging and immersive gameplay experiences.
Frequently Asked Questions
How do you create a random sequence of values that doesn’t repeat?
Creating non-repeating random sequences with constraints can be achieved using shuffling algorithms. By storing the last value generated and making multiple attempts to pick a new value if necessary, repeated results can be avoided.
Can you use random values to generate a random boolean value?
Boolean generation techniques can use random values to generate a random true or false outcome. Efficiencies of different randomization methods can be evaluated based on the likelihood of repeated results and the desired level of randomness.
How do you set a specific seed for Unity’s Random Class?
Using Random Seed in Unity is a way to control randomness in game development. The Init State function can be used to manually set the starting Seed for Unity’s Random Class, allowing for the recreation of specific random results.
What is the difference between using RGB and HSV when generating random colors?
RGB and HSV are both methods for random color generation in Unity, each with its own pros and cons. RGB allows for individual control of Red, Green, and Blue values, while HSV takes into account color theory with its Hue, Saturation, and Value elements. The choice ultimately depends on the desired outcome and the impact of color theory on the project.
How can you remove an option from a list after it has been randomly selected?
To remove an option from a list after it has been randomly selected, the option can be deleted from the list. Handling edge cases involves checking if the list is empty or if the option has already been removed to avoid removing duplicates.
Conclusion: Random Numbers In Unity Tutorial
In conclusion, this tutorial has provided a comprehensive overview of generating random numbers in Unity. We have explored the various methods available, including using the Random class and custom algorithms. Additionally, we have discussed the importance of seed values and how they can influence randomness in your game.
By understanding how to utilize random numbers effectively, you can add an element of unpredictability to your game mechanics, creating unique and engaging experiences for players.
We encourage you to share your thoughts, suggestions, or questions about this tutorial in the comments section below. Your feedback will help us improve and provide more valuable content in the future.
Don’t forget to visit our blog for more insightful articles on Unity development and other related topics. Explore further and enhance your skills as a game developer!
Thank you for reading, and we look forward to hearing from you!